Welcome to Nova 👋 (Beta)
Table of Contents
About
Nova is an opensource programming language built on node. The purpose of Nova is to make a pure psuedo-code language that is the perfect introduction into computer science. Completely built on node v12 and connected to npm packages, Nova is optimized for running on mac, linux and windows!
I made this project to make a pseudocode based language that simplified computer science. Nova's main purpose is to make it easier for non-programmers to learn the basics of coding. Using words such as "set" and "as" and "equals" makes it easier to follow and understand what is happening.
To get started go to usage and start your Nova journey today.
To support me, DanCodes, you can donate to my Patreon or just give this project a star :)
Usage
Install
npm i -g cli-nova
Run
nova [options] [file]
Example runs
nova test.ns
nova --verbose test.ns
Examples
// We recommend setting swift for language highlighting set variable as "hello"; // "Strings"output.log(variable); // Logging set two as 1 + 1; // Numbersset array as [1,2,3,4,5]; set chalk as include("chalk"); // Npm integrationoutput.log(chalk.red("Red text")); // Logs red output.log(12 / 2 % 2 + 1); // Logs 3 if two equals 2 then output.log("two is equal to 2"); if two isnot 2 then output.log("won't be logged") else output.log("two is not not equal to 2");
Keywords
Documentation
Variables
For variables we use two keywords, "set" and "as". All variable values are evaluated on initiation and stored in memory. They can be referenced at any time throughout the code and are global.
set hello as "world1";output.log("hello " + hello.slice(0, -1)); // output: hello world
Variables can also be set to npm modules and other files. Modules can be installed using npm.
set chalk as include("chalk");set path as include("path");set redText as chalk.red("red text"); output.log(redText); set package as include(path.resolve("./package.json"));output.log("Running v" + package.version);
Global Variables
Args
Description: Args is defined as arguments passed in the command line when starting nova.
Type: Array
Example:
// test.nsoutput.log; // Command line$ nova test.ns --test['--test']
Platform
Description: The platform the program is being run on, for example: linux, darwin and win32
Type: String
Example:
// test.nsoutput.log; // Command line on macbook$ nova test.nsdarwin
Process
Description: The process running containing information and functions to manipulate
Type: Object
Examples:
// test.nsset exitCode as 0;output.log;process.exit; // Command line$ nova test.nsProcess id is 12345
Nova
Description: File information and Nova information
Type: Object
Examples:
// test.nsoutput.log; // Command line$ nova test.ns
Tickers
Description: Intervals and timers that allow you to run something every certain time or after a certain time
Type: Function
Examples:
// test.nsset timer as
Include
Description: Include is an alias of node require and allows users to import npm modules and seperate files.
Type: Object
Examples:
// test.nsset chalk as include;output.log; // Command line$ nova test.nsI am red text :)
Output
Description: Output is an alias of node console and lets you output to the console
Type: Object
Examples:
// test.ns // This clears the outputoutput.log;output.error;output.info; // Command lineThis is regular logThis is an errorThis is some info
Author
👤 DanCodes dan@dancodes.online
- Website: https://dancodes.online
- Github: @dan-online
🤝 Contributing
Contributions, issues and feature requests are welcome!
Feel free to check issues page.
Show your support
Give a ⭐️ if this project helped you!
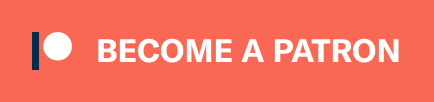
📝 License
Copyright © 2020 DanCodes dan@dancodes.online.
This project is MIT licensed.
This README was generated with ❤️ by readme-md-generator